Scraping Real-Time Data via Chrome DevTools Protocol
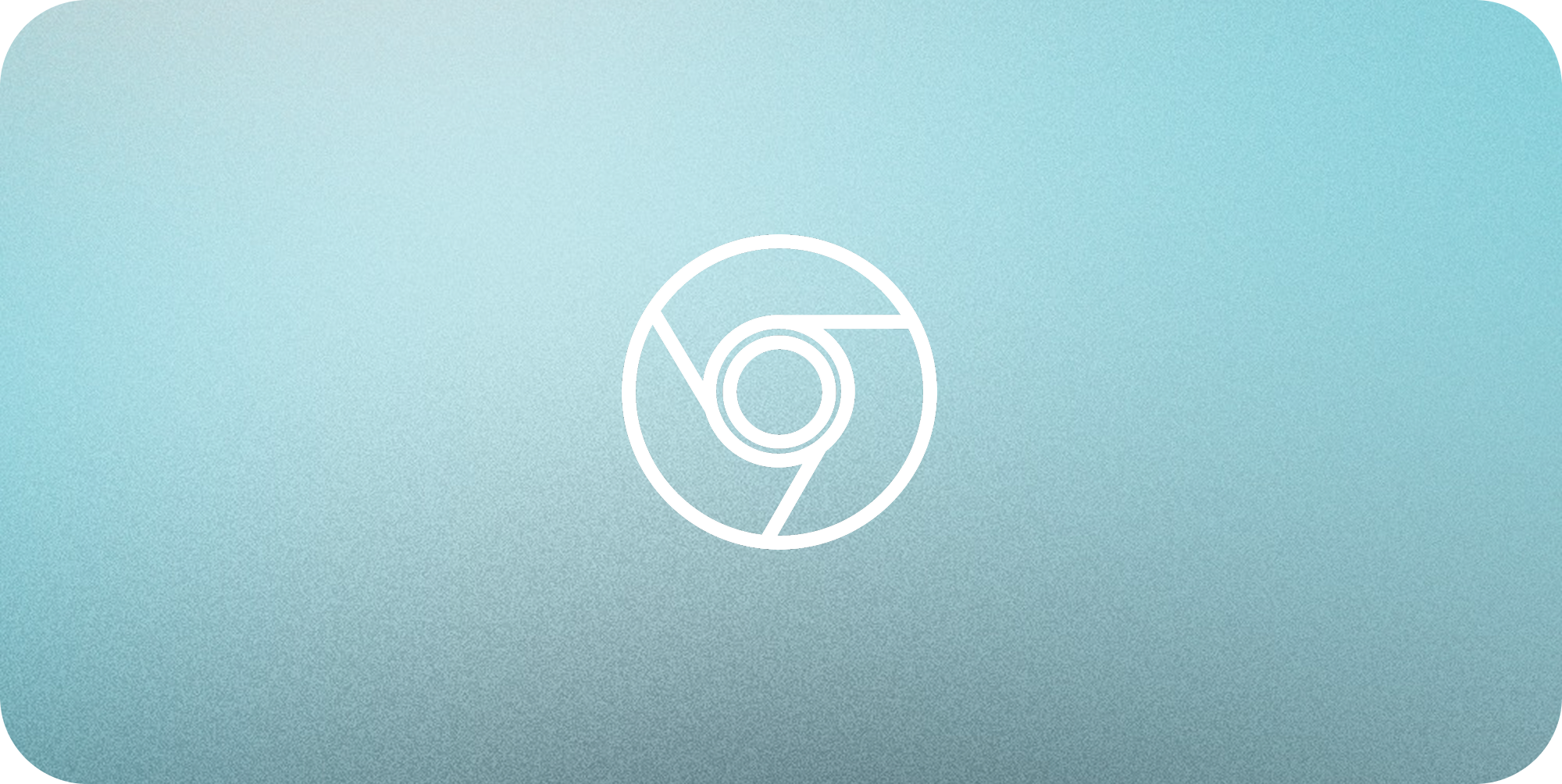
If you've spent any serious time scraping web data, you've probably hit that moment of pure frustration: the data you need isn't in the HTML. It's flowing through WebSockets, behind authentication walls, protected by Cloudflare, or fingerprinting your client six ways to Sunday.
The usual suspects—Puppeteer, Selenium, Beautiful Soup—they all fall short when it comes to WebSockets. You can automate the browser, sure, but actually intercepting and manipulating that real-time socket traffic? That's where things get messy.
The WebSocket Challenge
You could try connecting to the WebSocket with your own client but you'll end up running into these issues:
- Authentication tokens that expire or are tied to browser fingerprints
- Handshake failures when your custom client fails TLS fingerprinting
- Bot detection that triggers CAPTCHAs or silent blocks
- Reconnection logic & keeping the connection alive, some systems use proprietary formats instead of standard heartbeat
- Constantly changing endpoints that break your hardcoded connection strings
I spent weeks trying to reverse-engineer a trading platform's WebSocket API. Custom headers, handshake patterns, heartbeat messages—the works. Every time I thought I had it figured out, they'd change something.
Enter CDP: Chrome's Hidden Superpower
Chrome DevTools Protocol isn't new, but it's surprisingly underutilized due to its sparse documentation. Not many people know about it, despite being the same protocol that powers Chrome's own developer tools. Think about that for a second: the same pipe that lets you inspect network traffic in Chrome's DevTools can be tapped programmatically, yet it remains a hidden gem in the web scraping toolkit.
What makes CDP special is that it operates at a lower level than most automation tools. It doesn't just control the browser; it gives you hooks into the browser's internal communication systems. This means:
- You get raw access to WebSocket frames going in and out
- You bypass the need to implement custom WebSocket clients
- You inherit Chrome's TLS implementation, cipher suites, and fingerprint
- You get browser reconnection handling for free
In other words, you look exactly like a real browser because you ARE a real browser.
The Magic Interceptor Pattern
import { chromium } from "playwright";
async function captureWebSockets() {
const browser = await chromium.launch();
const context = await browser.newContext();
const page = await context.newPage();
// The CDP session is where the magic happens
const cdpSession = await context.newCDPSession(page);
await cdpSession.send('Network.enable');
// Listen for WebSocket messages
cdpSession.on('Network.webSocketFrameReceived', ({ response }) => {
const { payloadData } = response;
console.log(`Captured: ${payloadData}`);
// Do whatever you want with the data here...
});
await page.goto('https://example.com');
await new Promise(() => {}); // Keep running infinitely
}
That's it. Seriously. No need to manage WebSocket connections, handle reconnects, or deal with authentication flows. Your automation navigates the site normally, and CDP gives you a tap into the raw WebSocket traffic.
Closing thoughts
I hope you find this CDP approach useful in your web scraping projects. It's saved me countless hours of frustration and opened up data sources I previously thought were inaccessible. Happy scraping!
See all posts